.NET Security Considerations
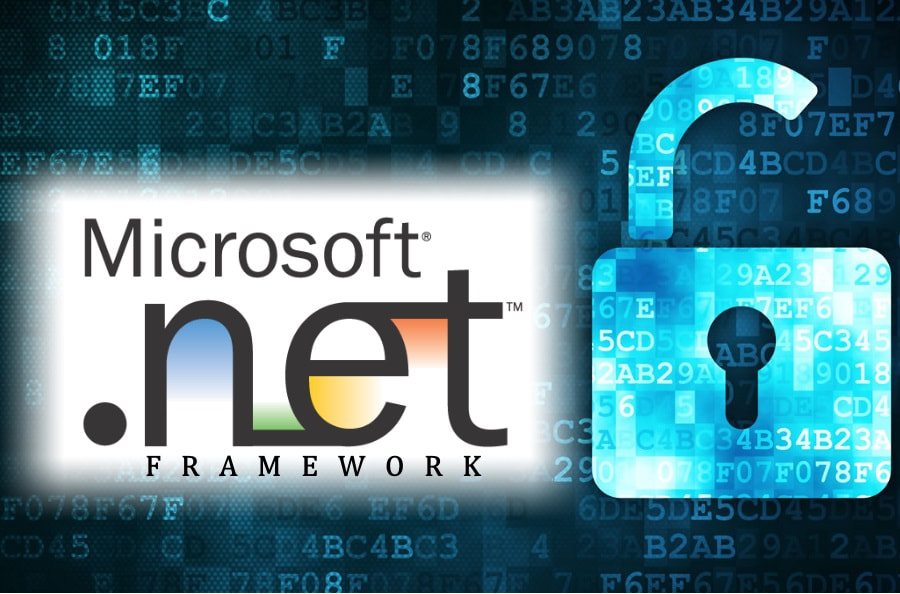
When it comes to developing software applications, security should always be a top priority. In the case of .NET development, it is no different. .NET offers a robust development platform, and within it, the C# programming language plays a significant role. However, it's essential to recognize that C# development in the .NET ecosystem also requires careful attention to security considerations. In my recent exploration of .NET development, I have come to appreciate the importance of incorporating strong security measures into .NET applications. In this blog, we will explore some of the most important security considerations that developers should keep in mind when working with .NET.
Input Validation
One of the most common security vulnerabilities in any software application is improper input validation. This occurs when an application does not properly filter or sanitize user input, which can lead to a variety of security issues, such as SQL injection attacks, cross-site scripting (XSS) attacks, and buffer overflows.
To prevent these types of attacks, it is important to always validate and sanitize user input. C# offers a variety of built-in functions and libraries that can help with input validation, such as the Regex class and the HttpUtility.HtmlEncode method. Here's an example of how to validate user input in C#:
string userInput = Request.Form["username"];
string sanitizedInput = SanitizeInput(userInput);
// Sanitization function to prevent XSS attacks
public string SanitizeInput(string input)
{
// Implement proper sanitization logic here
// ...
return sanitizedInput;
}
Secure Authentication and Authorization
It is essential to have secure authentication and authorization procedures to protect user identities and prevent illegal access. To enforce robust password hashing, secure session management, and role-based access control (RBAC), use built-in.NET capabilities such as ASP.NET Identity , IdentityServer, or OpenID Connect.
// Authenticating a user
bool isAuthenticated = await userManager.CheckPasswordAsync(user, password);
if (isAuthenticated)
{
// Grant access to authorized resources
}
else
{
// Handle authentication failure
}
Encryption and Hashing
Encryption and hashing are two important security techniques that can be used to protect sensitive data. Encryption involves converting data into an unreadable format, while hashing involves converting data into a fixed-length value that cannot be reversed. In .NET, developers can use the built-in System.Security.Cryptography namespace to implement encryption and hashing.
Secure Data Communication
Secure communication is important when transmitting sensitive data over the internet to prevent eavesdropping and data tampering. In .NET, developers can use the built-in System.Net.Security namespace to implement secure communication using SSL/TLS protocols. Here's an example of how to use SSL/TLS in .NET applications:
TcpClient client = new TcpClient("example.com", 443);
SslStream sslStream = new SslStream(client.GetStream(), false);
sslStream.AuthenticateAsClient("example.com");
Handling Sensitive Data
Maintaining confidentiality and integrity requires proper handling of sensitive data. Encrypt sensitive data with industry-standard methods, securely store secrets with Azure Key Vault or equivalent solutions, and adhere to best practices for security logging, data masking, and regulatory compliance.
// Encrypting and decrypting sensitive data
string encryptedData = EncryptionHelper.Encrypt(data, encryptionKey);
string decryptedData = EncryptionHelper.Decrypt(encryptedData, encryptionKey);
// Securely storing and retrieving secrets
string connectionString = KeyVault.GetSecret("DatabaseConnectionString");
Denial of Service (DoS) Prevention
Denial of Service (DoS) attacks are designed to disrupt the normal functioning of a system by overwhelming it with requests or traffic. Developers can use techniques like rate limiting and throttling to prevent DoS attacks.
Rate limiting involves limiting the number of requests that can be made to a system within a certain period of time. Throttling involves slowing down the rate at which requests are processed, in order to prevent the system from being overwhelmed. Here's an example of how to implement rate limiting in .NET applictions:
public class RateLimiter
{
private readonly int _maxRequests;
private readonly TimeSpan _timeSpan;
private readonly Dictionary> _requests;
public RateLimiter(int maxRequests, TimeSpan timeSpan)
{
_maxRequests = maxRequests;
_timeSpan = timeSpan;
_requests = new Dictionary>();
}
public bool CanMakeRequest(string ipAddress)
{
if (!_requests.ContainsKey(ipAddress))
{
_requests[ipAddress] = new List();
}
var requestsForIp = _requests[ipAddress];
requestsForIp.RemoveAll(t => t < DateTime.Now.Subtract(_timeSpan));
if (requestsForIp.Count >= _maxRequests)
{
return false;
}
requestsForIp.Add(DateTime.Now);
return true;
}
}
Conclusion
In conclusion, security is an essential aspect of software development. Developers should always be aware of the potential security vulnerabilities and take appropriate measures to prevent them. By following best practices for input validation, encryption and hashing, secure communication, and DoS prevention, developers can create secure and reliable software applications. Secure your .NET applications and build with confidence!